COMPLETE LOGIN SYSTEM IN PHP AND MYSQL
INDEX.PHP
<?php
session_start();
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>login system</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<nav>
<a href="#home"><img src="image/logo.jpg" id="logo"></a>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
<div class="login">
<form action="includes/login.inc.php" method="POST">
<input type="text" name="username" placeholder="enter your username or email">
<input type="password" name="passwd" placeholder="enter your password">
<button type="submit" name="login-btn" id="login-btn">LOGIN</button>
</form>
<?php
if(isset($_SESSION['id'])){
echo "
<form action='includes/logout.inc.php' method='POST'>
<button type='submit' id='signup' name='logout'>LOGOUT</button>
</form>
";
}
else{
echo "<a href='signup.php' class='btn'>SIGNUP</a>";
}
?>
</div>
</nav>
<div class="content">
<?php
if(isset($_SESSION['id'])){
echo "<h3>You are Logged In</h3>";
}
else{
echo "<h3>You are Logged Out</h3>";
}
?>
</div>
</body>
</html>
STYLE.CSS
*{
margin: 0;
padding: 0;
}
#logo{
padding: 6px 20px;
width: 40px;
height: 40px;
}
nav{
background-color: #f4f4f4;
width: 100%;
height: 100px;
}
nav ul{
display: inline-block;
}
nav ul li{
list-style: none;
float: left;
}
nav ul li a{
padding-left: 32px;
text-decoration: none;
color: #111;
font-size: 22px;
}
.login{
display: inline-block;
margin-left: 400px;
}
.login a{
display: inline-block;
background-color: #595454;
text-decoration: none;
}
.login button{
margin-top: 10px;
cursor: pointer;
background-color: #595454;
}
.login input{
height: 24px;
width: 120px;
}
#login-btn{
color: #fff;
background-color: blue;
padding: 8px 18px;
border-radius: 6px;
border: none;
cursor: pointer;
}
.btn{
outline: none;
border: none;
color: #fff;
background-color: blue;
padding: 6px 10px;
}
#signup{
background-color: #595454;
padding: 8px 18px;
color: #fff;
border: none;
}
.content{
padding: 30px;
background-color: #f4f4f4;
margin: 40px auto;
text-align: center;
}
.content h3{
background-color: #fff;
padding: 6px 2px;
}
.signup-form input{
height: 24px;
width: 120px;
margin: 12px;
}
.signup-form{
text-align: center;
margin: 60px auto;
background-color: #f4f4f4;
width:300px;
height: 400px;
}
#signup-btn{
color: #fff;
background-color: blue;
padding: 8px 18px;
border-radius: 6px;
border: none;
cursor: pointer;
}
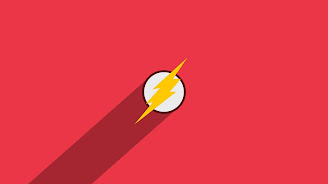
SIGNUP.PHP
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="css/style.css">
<title>signup form</title>
</head>
<body>
<div class="signup-form">
<h3>Signup</h3>
<?php
if(isset($_GET['error'])){
if($_GET['error']=="emptyfields"){
echo "<p style='color:red'>Please all Fields</p>";
}
else if($_GET['error']=="wronguname"){
echo "<p style='color:red'>Wrong Username</p>";
}
else if($_GET['error']=="wrongmail"){
echo "<p style='color:red'>Wrong Email</p>";
}
else if($_GET['error']=="checkpass"){
echo "<p style='color:red'>Both password must match</p>";
}
else if($_GET['error']=="usernametaken"){
echo "<p style='color:red'>Username already taken.. try different</p>";
}
}
else if(isset($_GET['signup']) == "success"){
echo "<p style='color:green'>SignUp Success.. Please Login</p>";
}
?>
<form action="includes/signup.inc.php" method="POST">
<input type="text" name="username" placeholder="Enter username"><br>
<input type="email" name="email" placeholder="Enter email"><br>
<input type="password" name="passwd" placeholder="enter password"><br>
<input type="password" name="re-passwd" placeholder="Re-enter password"><br>
<button type="submit" name="submit-btn" id="signup-btn">Signup</button>
</form>
</div>
</body>
</html>
SIGNUP.INC.PHP
<?php
require("db.php");
if(isset($_POST['submit-btn'])){
$username = mysqli_real_escape_string($conn,$_POST['username']);
$email = mysqli_real_escape_string($conn,$_POST['email']);
$password = mysqli_real_escape_string($conn,$_POST['passwd']);
$repeat_pass = mysqli_real_escape_string($conn,$_POST['re-passwd']);
if(empty($username) || empty($email) || empty($password) || empty($repeat_pass)){
header("Location: ../signup.php?error=emptyfields&uname=".$username."&mail=".$email);
exit();
}
else if(!preg_match("/^[a-zA-Z0-9]*$/",$username) && !filter_var($email,FILTER_VALIDATE_EMAIL))
{
header("Location: ../signup.php");
exit();
}
elseif(!preg_match("/^[a-zA-Z0-9]*$/",$username)){
header("Location: ../signup.php?error=wronguname&mail=".$email);
exit();
}
elseif(!filter_var($email,FILTER_VALIDATE_EMAIL)){
header("Location: ../signup.php?error=wrongmail&uname=".$username);
exit();
}
else if($password !== $repeat_pass){
header("Location: ../signup.php?error=checkpass&uname=".$username."&mail=".$email);
exit();
}
else{
$sql = "SELECT * FROM users WHERE username=?";
$stmt = mysqli_stmt_init($conn);
if(!mysqli_stmt_prepare($stmt,$sql)){
header("Location: ../signup.php?error=sqlerror");
exit();
}
else{
mysqli_stmt_bind_param($stmt,"s",$username);
mysqli_stmt_execute($stmt);
mysqli_stmt_store_result($stmt);
$result = mysqli_stmt_num_rows($stmt);
if($result > 0){
header("Location: ../signup.php?error=usernametaken");
exit();
}
else{
$sql = "INSERT INTO users(username,email,password) VALUES (?,?,?)";
$stmt = mysqli_stmt_init($conn);
if(!mysqli_stmt_prepare($stmt,$sql)){
header("Location: ../signup.php?error=sqlerror");
exit();
}
else{
$hash_pass = password_hash($password,PASSWORD_DEFAULT);
mysqli_stmt_bind_param($stmt,"sss",$username,$email,$hash_pass);
mysqli_stmt_execute($stmt);
header("Location: ../signup.php?signup=success");
exit();
}
}
}
}
mysqli_stmt_close($stmt);
mysqli_close($conn);
}
LOGIN.INC.PHP
<?php
session_start();
require("db.php");
if(isset($_POST['login-btn'])){
$username = mysqli_real_escape_string($conn,$_POST['username']);
$password = mysqli_real_escape_string($conn,$_POST['passwd']);
if(empty($username) ||empty($password)){
header("../index.php");
exit();
}
else{
$sql = "SELECT * FROM users WHERE username=? OR email=?";
$stmt = mysqli_stmt_init($conn);
if(!mysqli_stmt_prepare($stmt,$sql)){
header("Location: ../index.php?error=sqlerror");
exit();
}
else{
mysqli_stmt_bind_param($stmt,"ss",$username,$username);
mysqli_stmt_execute($stmt);
$result = mysqli_stmt_get_result($stmt);
if($row = mysqli_fetch_assoc($result)){
$passcheck = password_verify($password,$row['PASSWORD']);
if($passcheck == false){
header("Location: ../index.php?error=wrongpassword");
exit();
}
else if($passcheck == true){
$_SESSION['id'] = $row['id'];
$_SESSION['username'] = $row['username'];
header("Location: ../index.php?login=success");
exit();
}
else{
header("Location: ../index.php?error=wrongpassword");
exit();
}
}
else{
header("Location: ../index.php?error=nouser");
exit();
}
}
}
}
else{
header("Location: ../index.php");
exit();
}
LOGOUT.INC.PHP
<?php
session_start();
if(isset($_POST['logout'])){
session_unset();
session_destroy();
header("Location: ../index.php");
}
db.INC.PHP
<?php
$host = 'localhost';
$username = "root";
$password = "";
$dbname = "login";
$conn = mysqli_connect($host,$username,$password,$dbname);
if(!$conn){
die("connection fails".mysqli_connect_error());
}
?>
Betway Casino India – Review, Welcome Bonus and FS - JTM Hub
ReplyDeleteBetway Casino India review. The welcome bonus 상주 출장샵 is 100% match up to Rs.1,000, with 전라북도 출장안마 up to Rs.500 free bonus. 아산 출장샵 There is also the 울산광역 출장샵 welcome Rating: 4 · 여주 출장마사지 Review by JVMRubeo